Mesh generation
In the implementation of terrain mesh generation, a flexible and extensible
approach was taken by defining a base class, TerrainGenerator
, from which
specific terrain generation methods could be derived. This base class,
defined in terrain_generator.hpp
, serves as a common interface for generating
meshes. It includes a pure virtual method generate()
, which must be
implemented by any derived class to produce a mesh.
class TerrainGenerator
{
public:
using mesh_type = Mesh;
virtual std::unique_ptr<mesh_type> generate() = 0;
virtual ~TerrainGenerator() {};
};
Two primary subclasses were developed to handle different sources of elevation
data: LambdaGenerator
and GpsGenerator
. Both classes inherit from
TerrainGenerator
and share a common goal—creating a 3D mesh by initializing
its points and triangles. However, they differ in how they assign the z
values
(elevation) to the mesh points.
1. LambdaGenerator
The LambdaGenerator
class, defined in lambda_generator.hpp
and implemented
in lambda_generator.cpp
, allows for the creation of a mesh where the z
values are derived from a user-defined lambda function. This function is passed
to the constructor and is used to compute the elevation for each point in the
mesh grid.
class LambdaGenerator : public TerrainGenerator
{
public:
using fn_type = std::function<double(double, double)>;
LambdaGenerator(int meshSize, fn_type const &fn);
virtual ~LambdaGenerator();
std::unique_ptr<mesh_type> generate() override;
private:
int M_meshSize;
fn_type M_fn;
};
The mesh is generated on a regular grid, where each grid point’s elevation is
computed by applying the lambda function to its x
and y
coordinates.
After computing the elevations, the grid points are connected to form triangles,
resulting in a triangulated mesh.
Key steps in the LambdaGenerator::generate()
method include:
-
Grid points creation: The mesh grid is initialized, and each point is assigned a
z
value based on the provided lambda function. -
Triangle formation: Right triangles are created from adjacent grid points, ensuring a complete triangulated mesh.
-
Edges creation: Border edges are added to the mesh to define its boundary.
1.1. Surface of a sphere
The following lambda function:
where r
is the radius of the sphere and \((x_0, y_0)\) are the coordinates of
the center and z is the z-coordinate of the point on the sphere corresponding
to the input coordinates x and y.
This function translates in C++ like this:
auto fn = [=](double x, double y)
{
return std::sqrt(radius * radius - (x - centerX) * (x - centerX)
- (y - centerY) * (y - centerY));
};
and it results in the following mesh:
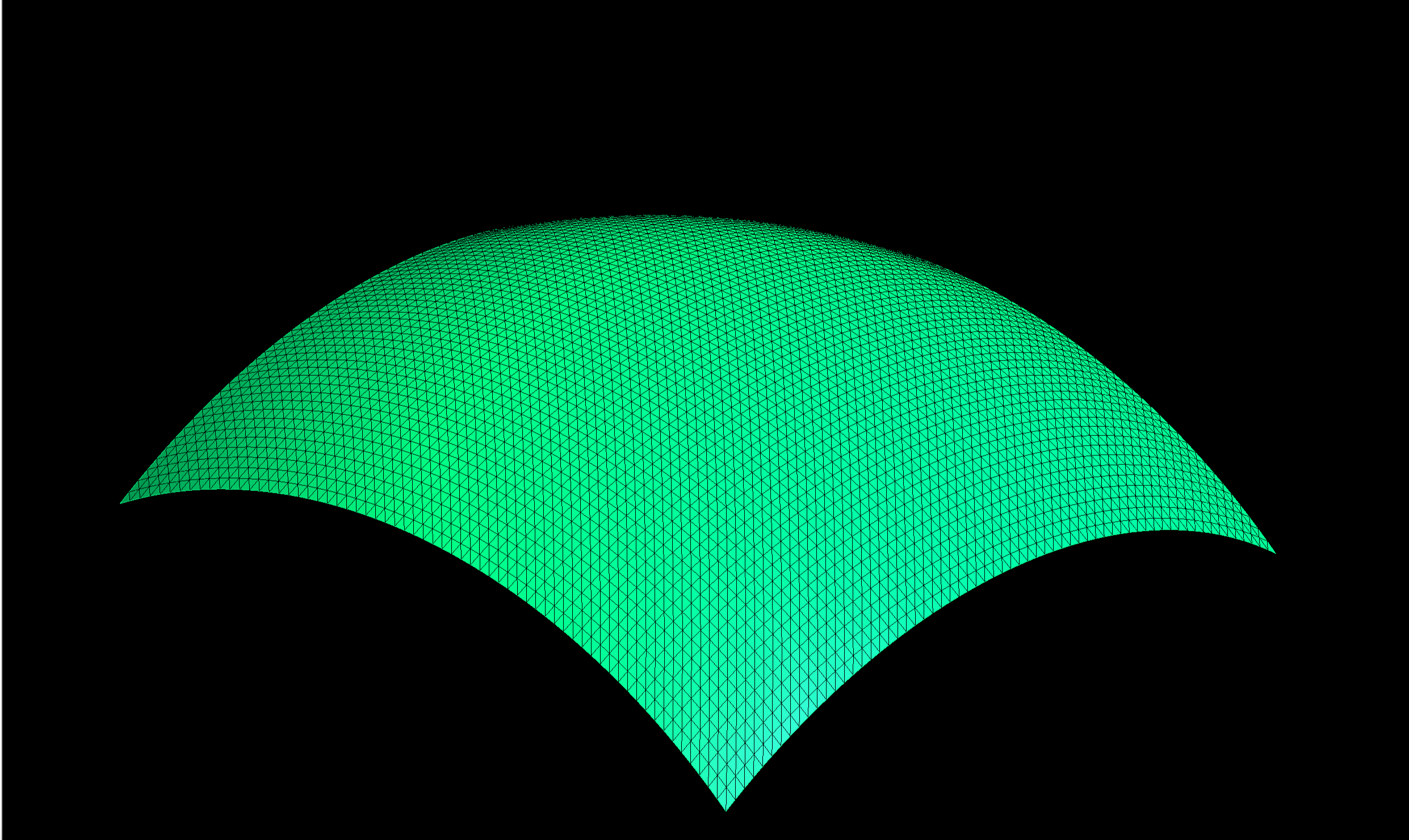
2. GpsGenerator
The GpsGenerator
class, defined in gps_generator.hpp
and implemented in
gps_generator.cpp
, generates a mesh where the z
values are obtained from
real-world elevation data. This data is fetched from a specified tile using
the Mapbox Terrain-RGB v1 API.
class GpsGenerator : public TerrainGenerator
{
public:
GpsGenerator(int meshSize, double latitude, double longitude, unsigned zoomLevel, std::string const &apiKey);
virtual ~GpsGenerator();
std::unique_ptr<mesh_type> generate() override;
private:
int M_meshSize;
double M_latitude;
double M_longitude;
unsigned M_zoomLevel;
std::string M_apiKey;
};
The process involves the following steps:
-
Tile downloading: The latitude, longitude, and zoom level specified in the configuration are used to determine the corresponding tile. The tile data is then downloaded using the
downloadTile()
function. -
Elevation data processing: The downloaded tile is decoded to extract elevation values. These values are used to initialize the
z
coordinates of the mesh points. -
Mesh construction: Similar to
LambdaGenerator
, the grid points are connected to form triangles, and border edges are added to define the mesh boundary.
Here is the resulting mesh, corresponding to the tile with coordinates (x, y) = (33809, 23527) at zoom level 16, which includes the location at longitude 5.7232 and latitude 45.1835, located in Grenoble, France:
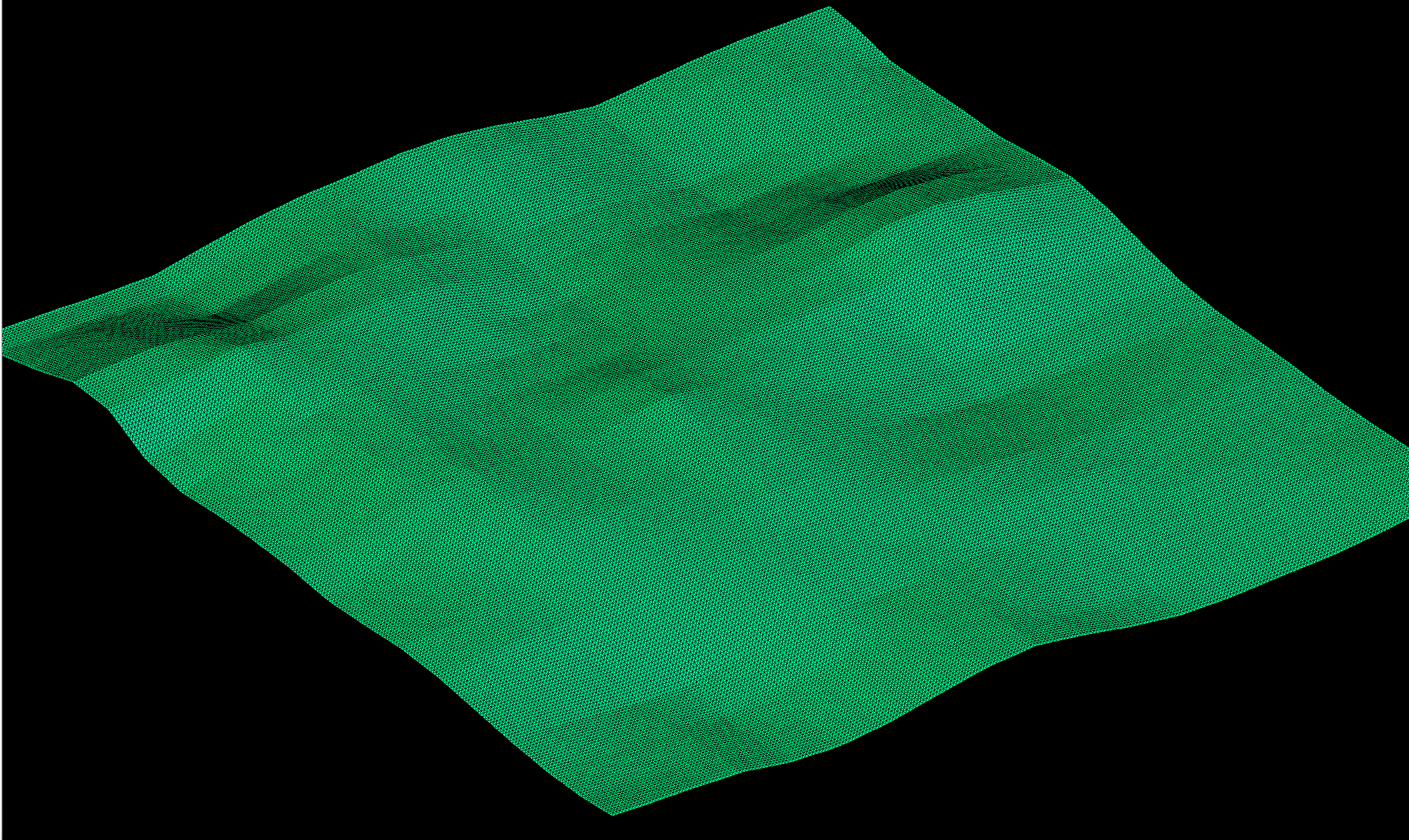
3. Common functionalities
Both LambdaGenerator
and GpsGenerator
share a common workflow for mesh
construction, with the primary difference being the source of the z
values:
-
Lambda function: In
LambdaGenerator
, thez
values are computed using a lambda function, providing flexibility in defining custom terrain shapes. -
GPS data: In
GpsGenerator
, thez
values are sourced from actual elevation data, enabling accurate representation of real-world terrains.
This modular approach, where the z
values are derived from either a lambda
function or real elevation data, allows the system to be extended easily
for different types of terrain generation methods while maintaining a
consistent structure for mesh construction.
References
-
[cemosis] Cemosis. Center for Modeling and Simulation in Strasbourg. 2024. (www.cemosis.fr)
-
[irma] IRMA. Institut de recherche mathématique avancée. 2024 (irma.math.unistra.fr)
-
[unistra] University of Strasbourg. 2024. (en.unistra.fr)
-
[numpex] PEPR Numpex. Priority Research Program and Equipment for Numerical Exascale computing. 2024. (numpex.org/numpex-program)
-
[exama] Exa-MA. Methods and Algorithms for Exascale. 2024. (numpex.org/exama-methods-and-algorithms-for-exascale)
-
[ktirio] Ktirio Urban Building application. Prud’homme Christophe. Expanding horizons: Ktirio and the urban building vision in Hidalgo2. October 2023. 2024. (github.com/orgs/feelpp/discussions/2167)
-
[hidalgo2] CoE Hidalgo2. HPC and big data technologies for global challenges. 2024. (www.hidalgo2.eu/about)
-
[ubm] CoE Hidalgo2. The Urban Building Model 2024. (www.hidalgo2.eu/urban-building-model)
-
[inria] Inria. National Institute for Research in Digital Science and Technology. 2024. (www.inria.fr/en)
-
[eea1] European Environment Agency. Greenhouse gas emissions from energy use in buildings in Europe. Octber 2023. 2024. (www.eea.europa.eu/en/analysis/indicators/greenhouse-gas-emissions-from-energy?activeAccordion=546a7c35-9188-4d23-94ee-005d97c26f2b)
-
[eea2] European Environment Agency. Accelerating the energy efficiency renovation of residential buildings — a behavioural approach. June 2023. 2024. (www.eea.europa.eu/publications/accelerating-the-energy-efficiency)
-
[ec1] European Commission. 2050 long-term strategy. 2024. (climate.ec.europa.eu/eu-action/climate-strategies-targets/2050-long-term-strategy_en#:~:text=Striving%20to%20become%20the%20world’s%20first%20climate%2Dneutral%20continent%20by%202050.&text=The%20EU%20aims%20to%20be,to%20the%20European%20Climate%20Law%20.)
-
[ec2] European Commission. The European Green Deal. 2024. (commission.europa.eu/strategy-and-policy/priorities-2019-2024/european-green-deal_en)
-
[ec3] European Commission. European Climate Law. 2024. (climate.ec.europa.eu/eu-action/european-climate-law_en)
-
[ubp] Urban Building Pilot. Prud’homme Christophe. CoE Hidalgo2 Urban Building Pilot at NumPEx workshop on Discretization@Exascale. November 2023. 2024. (github.com/orgs/feelpp/discussions/2188)
-
[eurohpc] EuroHPC JU. The European High Performance Computing Joint Undertaking. 2024. (eurohpc-ju.europa.eu/index_en)
-
[mapbox] Wikipedia contributors. Mapbox. Wikipedia, The Free Encyclopedia. August 2024. 2024. (en.wikipedia.org/wiki/Mapbox)
-
[mapbox-terrain-rgb] Mapbox. Mapbox Terrain-RGB v1. Mapbox Documentation. 2024. (docs.mapbox.com/data/tilesets/reference/mapbox-terrain-rgb-v1)
-
[mapbox-raster-tiles] Mapbox. Mapbox Raster Tiles API. Mapbox Documentation. 2024. (docs.mapbox.com/api/maps/raster-tiles)
-
[json-nlohmann] Lohmann, Niels. JSON for Modern C++. 2024. (json.nlohmann.me)
-
[curl] Stenberg, Daniel. cURL: A Command Line Tool and Library for Transferring Data with URLs. 2024. (curl.se)
-
[libpng] libpng: The Official PNG Reference Library. 2024. (www.libpng.org/pub/png/libpng.html)
-
[cgal] CGAL: The Computational Geometry Algorithms Library. 2024. (www.cgal.org)
-
[stl] STL (STereoLithography) File Format Specification. 2024. (www.fabbers.com/tech/STL_Format)
-
[gmsh] Geuzaine Christophe, and Jean-François Remacle. Gmsh: A 3D Finite Element Mesh Generator with Built-in Pre- and Post-Processing Facilities. Version 4.10, 2024. (gmsh.info)
-
[msh] MSH: The Gmsh Mesh File Format. 2024. (gmsh.info/doc/texinfo/gmsh.html#MSH-file-format)
-
[img:lat-lon] Latitude and Longitude. BBC Bitesize. 2024. (www.bbc.co.uk/bitesize/guides/ztqtyrd/revision/1)
-
[world-geodetic-system] Wikipedia contributors. World Geodetic System. Wikipedia, The Free Encyclopedia. 2024. (en.wikipedia.org/wiki/World_Geodetic_System)
-
[marcator-projection] Wikipedia contributors. Mercator projection. Wikipedia, The Free Encyclopedia. 2024. (en.wikipedia.org/wiki/Mercator_projection)
-
[web-marcator-projection] Wikipedia contributors. Web Mercator projection. Wikipedia, The Free Encyclopedia. 2024. (en.wikipedia.org/wiki/Web_Mercator_projection)
-
[cdt1] Wikipedia contributors. Constrained Delaunay triangulation. Wikipedia, The Free Encyclopedia. 2024. (en.wikipedia.org/wiki/Constrained_Delaunay_triangulation)
-
[cdt2] L. Paul Chew. Constrained Delaunay Triangulations. Dartmouth College. 1987. 2024. (www.cs.jhu.edu/~misha/Spring16/Chew87.pdf)
-
[dt] Wikipedia contributors. Delaunay triangulation. Wikipedia, The Free Encyclopedia. 2024. (en.wikipedia.org/wiki/Voronoi_diagram)
-
[voronoi] Wikipedia contributors. Voronoi diagram. Wikipedia, The Free Encyclopedia. 2024. ()
-
[tiled-web-map] Wikipedia contributors. Tiled web map. Wikipedia, The Free Encyclopedia. 2024. (en.wikipedia.org/wiki/Tiled_web_map)
-
[img:tiles-coordinates] XYZ Tiles coordinate numbers. Wikipedia, The Free Encyclopedia. 2024. (en.wikipedia.org/wiki/File:XYZ_Tiles.png)
-
[img:tiled-web-map] Tiled Web Map. Wikipedia, The Free Encyclopedia. 2024. (en.wikipedia.org/wiki/Tiled_web_map#/media/File:Tiled_web_map_Stevage.png)
-
[img:dt] Delaunay triangulation. Wikipedia, The Free Encyclopedia. 2024. (en.wikipedia.org/wiki/Delaunay_triangulation#/media/File:Delaunay_circumcircles_vectorial.svg)
-
[img:dt-centers] Delaunay triangulation with all the circumcircles and their centers. Wikipedia, The Free Encyclopedia. 2024. (en.wikipedia.org/wiki/Delaunay_triangulation#/media/File:Delaunay_circumcircles_centers.svg)
-
[img:dt-voronoi] Delaunay triangulation and its Voronoi diagram. Wikipedia, The Free Encyclopedia. 2024. (en.wikipedia.org/wiki/Delaunay_triangulation#/media/File:Delaunay_Voronoi.svg)
-
[img:constrained-mesh] Pierre Alliez, Senior Researcher and Team Leader at Inria, Image provided during personal communication. 2024.
-
[img:constrained-refined-mesh] Pierre Alliez, Senior Researcher and Team Leader at Inria, Image provided during personal communication. 2024.